JAVA QUESTIONS
Happy reading...😊
1.What are the differences between C++ and Java? Which one do you think is better and why?
Ans:Both are based on OOPS concept. Following are the basic differences –
When asked your opinion on
which is better, there is no right or wrong answer. You can say what you like
about C++ or Java more. For example, I don’t like pointers and Java doesn’t
have it, so I can vote for Java. On the other hand, C++ supports operator overloading
and passing by reference while Java doesn’t, so I can like C++ more because of
this flexibility. This question is just to test if you can analyze and weigh
the pros and cons of each.
2.How is polymorphism implemented in Java?
Ans:Method overloading or static polymorphism
That means a method with the same name can have different number of parameters. Based on the parameter list, the appropriate method will be called. For example,
Method overloading
print(String name){
//code
}
print(int marks, String name){
//code
}
print(String[] subjects, String name){
//code
}
// in the main program,
if(subjects.length >0){
print(String[] subjects, String name);
}else if(marks>0){
print(int marks, String name);
}else
print(String name);
Overriding or dynamic polymorphism
This is the case when a child class extends parent class. During run time when the object is created, the appropriate method will be created. You can take any example –
class Book{
void turnpage(){
System.out.println(“You are in the next page!”);
}
}
class BookShelf extends Book{
void turnpage(){
System.out.println(“The book is taken, You are in the new page!”);
}
}
In the main class,
public static void main(String[] args) {
Book b = new BookShelf();
b.turnpage();
This means that the method turnpage() is overridden by the child class BookShelf at runtime.
3.What is the difference between stack and heap memory?
Ans:Heap –
- JRE uses it to allocate memory for objects and JRE classes.
- Garbage collection is done on heap memory
- Objects created on heap are accessible globally.
Stack –
- Short term references like the current thread of execution
- References to heap objects are stored in stack
- When a method is called, a new memory block is created. Once the method gets executed, the block is used by the next program.
Stack memory size is smaller compared to heap memory.
4.What is ‘null’ and how is memory allocation done for null objects?
Ans:When a non-primitive variable doesn’t point or refer to any object, it is called null.
- String str = null; //declaring null
- if(str == null) //Finding out if value is null
- int length = str.length();//using a null value will throw NullPointerException.
5.What are the advantages of a thread? How does the multithreading look like?
Ans:These are the following advantages of a Thread:
Multiprocessing and multithreading
One process may contain more than one thread and execute simultaneously is known as multi-threading.
- It provides efficient communication.
- It minimizes the context switching time.
- By using thread, we can get the concurrency within a process.
- Thread allows utilization of multiprocessor architectures to a greater scale and efficiency.
- A process which executes multiple threads simultaneously is known as multithreading.
- A thread is a lightweight sub-process, the smallest unit of processing.
Multiprocessing and multithreading
One process may contain more than one thread and execute simultaneously is known as multi-threading.
6.What is the difference between Abstract classes and interface?
Ans:Java interface should be implemented using the keyword "implements"; A Java abstract class should be extended using the keyword "extends."
An Interface can extend interface only; Abstract class have two properties, it can extend only one java class but implement more than one interface at a time.
7. What is the logic behind reverse of a string in java?
Ans:The reverse of a string:
import java.util.*;
class ReverseString
{
public static void main(String parvez[])
{
String original, reverse= " ";
Scanner in=new scanner(system.in);
System.out.println("Enter the string: ");
original= in.nextLine();
int length=original.Length();
for(i=length-1;i>=0;i--)
reverse=reverse+original.charAt(i);
System.out.println("Reversed string: " +reverse);
}
}
Output:
Enter the string:parvez
Reversed string:zevrap
8.What is the major difference between yield () and sleep ()?
Ans:Sleep () method in Java has two variants one which takes millisecond as sleeping time while other which takes both millisecond and nanosecond for the sleeping duration.
Yield () method pause all the currently executing thread, and give a chance to execute those threads or processes that need to be run. The current thread will continue to run again if no other thread is available to execute.
9.What Are Wrapped Classes?
Ans:Wrapper class in Java provides the mechanism to convert primitive into object and object into primitive. The eight classes of the java.lang package is known as wrapper classes in java.
The list of eight wrapper classes is given below:
Primitive Type
Wrapper class
Boolean
Boolean
Char
Character
Byte
Byte
Short
Short
Int
Integer
Long
Long
Float
Float
Double
Double
10.What is garbage collection? Does it guarantee that
a program will not run out of memory?
Ans:Garbage Collection is a process of reclaiming the unused runtime memory automatically. i.e., it is a way to destroy the unused objects.
To do so, we were using free () function in the C language and delete () in C++. But, in Java, it is performed automatically. So, java provides better memory management.
In some cases, programs use up memory resources faster than they are garbage collected. We can also create objects "without new keyword" in programs that are not subject to garbage collection. That's why garbage collection doesn't guarantee that a program will not run out of memory.
Primitive Type
Wrapper class
Boolean
Boolean
Char
Character
Byte
Byte
Short
Short
Int
Integer
Long
Long
Float
Float
Double
Double
11.Suppose there are two processes A & B,is there a way that they can send messages to each other?
Ans:Java processes can communicate with each other using threads.Threads enable multi-tasking by completing more than one tasks in parallel without interfering in each others data.
12.What are JVM and JIT?
Ans:Java code can be executed anywhere because the code is converted from Java bytecodes into the native code of a machine.This is achieved by the JVM(Java Virtual Machine).
A file is compiled only for the first time until it is changed later.If the byte-code is not changed,JVM,the intelligent processor will not waste time compiling such files again.This is called JIT or Just In Time compilation.
13.Which takes more memory-float or Float?
Ans:float is a native data type whereas Float is a class.A Float object will always take more memory than float variables as there are metadata overheads with the objects.14.What are the different ways to handle an exception?
Ans:1.Wrap the desired code in try block followed by a catch/finally to catch the exception.2.Declare the throws clause with exception that the code is expected to throw as an indication to the calling method to take care of it.
15.Why Java is not 100% object-oriented?
Ans:Java is not 100% object oriented because it makes use of eight primitive data types such as these boolean ,byte ,char ,int ,float ,double ,long ,short which are not objects.16. Is delete, next, main, exit or null are keywords in java?
Ans: Only null is a keyword here, rest all are methods
17.What are the advantage of package in java?
Ans:1)Package avoid name clashes
2)Package can provide easier access control
3)Package can provides the re-usability of the code
4)We can create our own package or can use already defined package
18.What is the output of the code?
class Test
{
public static void main (String args[])
{
System.out.println(10 + 20 + "codeland");
System.out.println("codeland" + 10 + 20);
}
}
{
public static void main (String args[])
{
System.out.println(10 + 20 + "codeland");
System.out.println("codeland" + 10 + 20);
}
}
Ans: 30codeland
codeland1020
First case:10 and 20 are taken as numbers(before the string)
Second case:10 and 20 are taken as strings(after the string)
19.Which are the two subclasses under Exception class?
Ans:The Exception class has two main subclasses: IOException class and RuntimeException class .20.When a byte datatype is used?
Ans:This datatype is used to save space in large arrays,mainly in place of integers,since a byte is four times smaller than an int.21.What is the difference between throw and throws?
Ans:Throw is used to trigger an exception where as throws is used in declaration of exception.Without throws ,Checked exception cannot be handled where as checked exception can be propagated with throws.
22.What are the ways in which a thread can enter the waiting state?
Ans:A thread can enter the waiting state by invoking its sleep() method, by blocking on IO, by unsuccessfully attempting to acquire an object's lock, or by invoking an object's wait() method. It can also enter the waiting state by invoking its (deprecated) suspend() method.
23.What is the difference between the methods sleep() and wait()?
Ans:The code sleep(2000); puts thread aside for exactly two seconds. The code wait(2000), causes a wait of up to two second. A thread could stop waiting earlier if it receives the notify() or notifyAll() call. The method wait() is defined in the class Object and the method sleep() is defined in the class Thread.24.What is error and Exception?
Ans:An error is an irrecoverable condition occurring at runtime. Such as OutOfMemory error. Exceptions are conditions that occur because of bad input etc. e.g. FileNotFoundException will be thrown if the specified file does not exist.
25.What is synchronization?
Ans:Synchronization is the capability to control the access of multiple threads to shared resources. synchronized keyword in java provides locking which ensures mutual exclusive access of shared resource and prevent data race.
26.Explain Map and their types in Java.
Ans: A Java Map is an object that maps keys to values. It can’t contain duplicate keys and each key can map to only one value. In order to determine whether two keys are the same or distinct, Map makes use of the equals() method. There are 4 types of Map in Java, described as follows:
- HashMap - It is an unordered and unsorted map and hence, is a good choice when there is no emphasis on the order. A HashMap allows one null key and multiple null values and doesn’t maintain any insertion order.
- HashTable – Doesn’t allow anything null and has methods that are synchronized. As it allows for thread safety, the performance is slow.
- LinkedHashMap – Slower than a HashMap but maintains insertion order and has a faster iteration.
- TreeMap – A sorted Map providing support for constructing a sort order using a constructor.
27.What are the differences between the constructors and methods?
Ans:
Java Constructor
|
Java Method
|
A constructor is used to initialize the state of
an object.
|
A method is used to expose the behaviour of an
object.
|
A constructor must not have a return type.
|
A method must have a return type.
|
The constructor is invoked implicitly.
|
The method is invoked explicitly.
|
The Java compiler provides a default constructor
if you don't have any constructor in a class.
|
The method is not provided by the compiler in any
case.
|
The constructor name must be same as the class
name.
|
The method name may or may not be same as class
name.
|
28.Why pointers are not used in Java?
Ans:Java doesn’t use pointers because they are unsafe and increases the complexity of the program. Since, Java is known for its simplicity of code, adding the concept of pointers will be contradicting. Moreover, since JVM is responsible for implicit memory allocation, thus in order to avoid direct access to memory by the user, pointers are discouraged in Java.
29.Explain Generics in Java ?
Ans: Generics are a facility of generic programming that were added to the Java programming language in 2004 within J2SE5.0. They allow "a type or method to operate on objects of various types while providing compile-time type safety.
30.What does the "String[ ] args" in a Java main method actually mean?
Ans:String[] args in Java is an array of strings which stores arguments passed by command line while starting a program. All the command line arguments are stored in that array.
example:
- public class Test {
- public static void main(String[] args) {
- for(String str : args) {
- System.out.println(str);
- }
- }
- }
If you compile it and run it as following:
java Test This is a test of command line arguments.
The following output is generated:
Thisisatestofcommandlinearguments.
31.Can we overload the main() method in Java?
Ans:Yes, we can have multiple methods with the same name as main inside a single class. However, JVM will consider the method with the syntax
public static void main(String args)
as the main()
method.32.Why is Java main() method static?
Ans:Before getting into the answer, let us see what is the use of declaring a method as static? Generally in Java, a method is invoked using an object created for the class (in which the same method is written). But when a method is declared as static, it can be invoked without the use of the object, i.e., directly using the class.
We all know that the execution of a Java program starts from a main() method. Since the main() method has to be invoked before invoking any other method, it is always declared as static in Java.
33.What are Array, List Interface, ArrayList and Vector?
Ans:Array:
Generally, Array is a collection of similar type of elements that are arranged in adjacent memory addresses. In Java, Array is an object which contains similar types of elements. By default, the size of these elements stored in an array cannot be changed. i.e, you will give a size to the array and you cannot expand or reduce it later. If you want to change the size of the array, you have to create a new array and copy the data that you want. But this process is tedious. But, in Platforms such as Java 6 and Java 7, built-in classes like ArrayList are introduced.
List Interface:
The list interface is the ordered collection of objects in which you can store duplicate values. In list interface, you can insert elements in desired positions as it has insertion and positional access.
ArrayList:
The class ArrayList is an array-based implementation of the List interface. All the elements of the ArrayList are stored in a Java array and hence, it has fixed size in the beginning. For example, an array list by the name of xyz is of the size m. In the beginning, the array xyz is capable of storing a maximum of m elements. But, when the (m+1)th element is added to the array, the size of the array will be increased by 50% of its original size automatically. This is done by acquiring a bigger array and copying all the elements of the current array to the bigger array.
Vector:
Like ArrayList, Vector also implements List interface and uses insertion order. But, unlike ArrayList, Vector is synchronized. Even though the adding, searching, updating and deleting of arrays are not carried out satisfactorily because of the synchronization, the vectors can grow and shrink as required to accommodate elements. In the beginning, the using this vector class object will create an initial capacity of m. If the (m+1)th element is added, the size of the vector is doubled.
Declaring the vector in its default form as in Vector xyz = new Vector(); will create 10 initial capacity. In case of Vector xyz = new Vector(int size, int incr); the initial capacity is specified by the size and the increment of the size is specified by incr.
34.What is a Servlet?
Ans: Servlets and applets came into the picture as soon as the web was used for delivering services. This is because the service providers recognized the need for a more dynamic content. Hence, at first, the applets was developed. It completely focused on using the client Platform to provide the dynamic experience. At the same time, the servlets are developed to give the dynamic experience from the server platform.
Java servlet is a Java program that extends the capabilities of a server. A Java Servlet container provides lifecycle management which is a single process to share and manage application-wide resources and interaction with a Web server. The primary use of the Java Servlet is to define a robust mechanism for sending content to a client as according to the definition of the Client/Server model. Also, Servlets natively supports HTTP.
It processes the input from client side and generates the response in terms of the HTML page, Applets or Javascript. The lifecycle of a servlet consists of init( ), service( ), and destroy( ).
35.What are the similarities between C++ and Java?
Ans:Java, unlike C++, doesn’t have pointers, operator overloading, typedef keyword, define keyword, structures, unions, enumeration, functions, goto statements, templates, and default parameters.
There is no automatic coercion in Java, unlike C++. Coercion is to convert one data type to another. In Java, coercion is explicitly made and is performed using code instructions.
Unlike C++, Java has garbage collection and multithread support.
Like C++, it also supports internationalization and exception handling.
36.What is the difference between string builder and string buffer in Java?
Ans:Both string builder and string buffer are two different classes used to create mutable strings in Java. However, they too have some differences.
String builder
|
String buffer
|
StringBuilder
is non-synchronized (not thread-safe). This means two threads can call the
methods of StringBuilder class simultaneously.
|
StringBuffer
is synchronized (thread-safe). This means two threads cannot call the methods
of StringBuffer class simultaneously.
|
Since
StringBuilder is non-synchronized, it is more efficient than StringBuffer.
|
StringBuffer
is less efficient than StringBuilder.
|
37.How will you create an object for a class without the use of 'new' keyword?
Ans:You can create an object without the use of new keyword using the below techniques.
1) Using newInstance
We can use
newInstance()
method to create an object for a class without the use of new
keyword as shown below.class Flower {
void print() {
System.out.println("Flowers are beautiful");
}
}
class Main {
public static void main(String args[]) throws ClassNotFoundException, InstantiationException, IllegalAccessException {
Class cls = Class.forName("Flower");
Flower f = (Flower) cls.newInstance();
f.print();
}
}
Class.forName
just loads the class in Java. It doesn't create any object. To create an object, you have to use the newInstance()method of the class named 'Class'.38.What is a Singleton class?
Ans:A singleton class in Java is a class that has only one object at a time. This class is used when there is a limitation on the number of objects of a class.To create a singleton class,
- Make the constructor as private
- Write a static method that has a return type object of this singleton class
39.Does importing a package import its sub-packages as well in Java?
Ans:No. In Java, when we import a package, its sub-packages will not get imported automatically. We need to do it explicitly whenever required.
40. What is Fork/Join framework?
Ans:This is not a new concept but is now used in multiple ways since the release of Java 8. Fork-Join breaks the task at hand into mini-tasks until the mini-task is simple enough that it can be solved without further breakups. It’s like a divide-and-conquer algorithm. One important concept to note in this framework is that ideally no worker thread is idle. They implement a work-stealing algorithm in that idle workers steal the work from those workers who are busy.
41.What is the platform?
Ans:A platform is the hardware or software environment in which a piece of software is executed. There are two types of platforms, software-based and hardware-based. Java provides the software-based platform.
42.What is this keyword in java?
Ans:The this keyword is a reference variable that refers to the current object. There are the various uses of this keyword in Java. It can be used to refer to current class properties such as instance methods, variable, constructors, etc. It can also be passed as an argument into the methods or constructors. It can also be returned from the method as the current class instance.
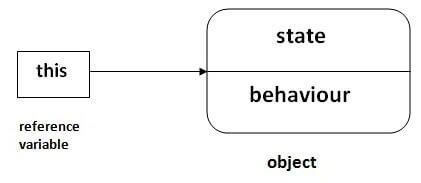
43.What is the output of the following Java program?
1. public class Test
2.
3. public static void main (String args[])
4. {
5. String a = new String("Sharma is a good player");
6. String b = "Sharma is a good player";
7. if(a == b)
8. {
9. System.out.println("a == b");
10. }
11. if(a.equals(b))
12. {
13. System.out.println("a equals b");
14. }
15. }
|
Output:
a equals b
a equals b
Explanation
The operator == also check whether the references of the two string objects are equal or not. Although both of the strings contain the same content, their references are not equal because both are created by different ways(Constructor and String literal) therefore, a == b is unequal. On the other hand, the equal() method always check for the content. Since their content is equal hence, a equals b is printed.
44.What are autoboxing and unboxing? When does it occur?
Ans:The autoboxing is the process of converting primitive data type to the corresponding wrapper class object, eg., int to Integer. The unboxing is the process of converting wrapper class object to primitive data type. For eg., integer to int. Unboxing and autoboxing occur automatically in Java. However, we can externally convert one into another by using the methods like valueOf() or xxxValue().
It can occur whenever a wrapper class object is expected, and primitive data type is provided or vice versa.- Adding primitive types into Collection like ArrayList in Java.
- Creating an instance of parameterized classes ,e.g., ThreadLocal which expect Type.
- Java automatically converts primitive to object whenever one is required and another is provided in the method calling.
- When a primitive type is assigned to an object type.
45.How to create packages in Java?
Ans:If you are using the programming IDEs like Eclipse, NetBeans, MyEclipse, etc. click on file->new->project and eclipse will ask you to enter the name of the package. It will create the project package containing various directories such as src, etc. If you are using an editor like notepad for java programming, use the following steps to create the package.
- Define a package package_name. Create the class with the name class_name and save this file with your_class_name.java.
- Now compile the file by running the following command on the terminal.
- javac -d . your_class_name.java The above command creates the package with the name package_name in the present working directory.
- Now, run the class file by using the absolute class file name, like following.
- java package_name.class_name
46.Why is multiple inheritance not supported in java?
Ans:To reduce the complexity and simplify the language, multiple inheritance is not supported in java. Consider a scenario where A, B, and C are three classes. The C class inherits A and B classes. If A and B classes have the same method and you call it from child class object, there will be ambiguity to call the method of A or B class.
Since the compile-time errors are better than runtime errors, Java renders compile-time error if you inherit 2 classes. So whether you have the same method or different, there will be a compile time error.
|
Compile time error.
47.What is String Pool?
Ans:String pool is the space reserved in the heap memory that can be used to store the strings. The main advantage of using the String pool is whenever we create a string literal; the JVM checks the "string constant pool" first. If the string already exists in the pool, a reference to the pooled instance is returned. If the string doesn't exist in the pool, a new string instance is created and placed in the pool. Therefore, it saves the memory by avoiding the duplicacy.
48.What are the steps to connect to the database in java?
Ans:The following steps are used in database connectivity.
- Registering the driver class: The forName() method of the Class class is used to register the driver class. This method is used to load the driver class dynamically. Consider the following example to register OracleDriver class.
- Creating connection: The getConnection() method of DriverManager class is used to establish the connection with the database. The syntax of the getConnection() method is given below.
- 1) public static Connection getConnection(String url)throws
- SQLException
- 2) public static Connection getConnection(String url,String name,String password)
- throws SQLException
- Creating the statement: The createStatement() method of Connection interface is used to create the Statement. The object of the Statement is responsible for executing queries with the database.
- Statement stmt=
- con.createStatement();
- Executing the queries:
- ResultSet rs=stmt.executeQuery("select * from emp");
- while(rs.next()){
- System.out.println(rs.getInt(1)+" "+rs.getString(2));
- }
- Closing connection: By closing connection, object statement and ResultSet will be closed automatically. The close() method of Connection interface is used to close the connection.
- con.close();
1. Class.forName("
oracle.jdbc.driver.OracleDriver ");
|
Consider the following example to establish the connection with the Oracle database.
public Statement createStatement()throws SQLException
consider the following example to create the statement object
The executeQuery() method of Statement interface is used to execute queries to the database. This method returns the object of ResultSet that can be used to get all the records of a table.
Syntax of executeQuery() method is given below.
public ResultSet executeQuery(String sql)throws SQLException
|
Example to execute the query
However, to perform the insert and update operations in the database, executeUpdate() method is used which returns the boolean value to indicate the successful completion of the operation.Syntax of close() method is given below.
public void close()throws SQLException
Consider the following example to close the connection.
49.What is the role of the JDBC DriverManager class?
Ans:The DriverManager class acts as an interface between user and drivers. It keeps track of the drivers that are available and handles establishing a connection between a database and the appropriate driver. The DriverManager class maintains a list of Driver classes that have registered themselves by calling the method DriverManager.registerDriver().
50.What is the difference between Java 7 and Java 8?
Ans:
51.What is classloader?
Ans: Classloader is a subsystem of JVM which is used to load class files. Whenever we run the java program, it is loaded first by the classloader. There are three built-in classloaders in Java.
- Bootstrap ClassLoader: This is the first classloader which is the superclass of Extension classloader. It loads the rt.jar file which contains all class files of Java Standard Edition like java.lang package classes, java.net package classes, java.util package classes, java.io package classes, java.sql package classes, etc.
- Extension ClassLoader: This is the child classloader of Bootstrap and parent classloader of System classloader. It loads the jar files located inside $JAVA_HOME/jre/lib/ext directory.
- System/Application ClassLoader: This is the child classloader of Extension classloader. It loads the class files from the classpath. By default, the classpath is set to the current directory. You can change the classpath using "-cp" or "-classpath" switch. It is also known as Application classloader.
52.What are the various access specifiers in Java?
Ans:In Java, access specifiers are the keywords which are used to define the access scope of the method, class, or a variable. In Java, there are four access specifiers given below.
- Public The classes, methods, or variables which are defined as public, can be accessed by any class or method.
- Protected Protected can be accessed by the class of the same package, or by the sub-class of this class, or within the same class.
- Default Default are accessible within the package only. By default, all the classes, methods, and variables are of default scope.
- Private The private class, methods, or variables defined as private can be accessed within the class only.
52.Why is Inheritance used in Java?
Ans:There are various advantages of using inheritance in Java that is given below.
- Inheritance provides code reusability. The derived class does not need to redefine the method of base class unless it needs to provide the specific implementation of the method.
- Runtime polymorphism cannot be achieved without using inheritance.
- We can simulate the inheritance of classes with the real-time objects which makes OOPs more realistic.
- Inheritance provides data hiding. The base class can hide some data from the derived class by making it private.
- Method overriding cannot be achieved without inheritance. By method overriding, we can give a specific implementation of some basic method contained by the base class.
54.What is composition?
Ans:Holding the reference of a class within some other class is known as composition. When an object contains the other object, if the contained object cannot exist without the existence of container object, then it is called composition. In other words, we can say that composition is the particular case of aggregation which represents a stronger relationship between two objects. Example: A class contains students. A student cannot exist without a class. There exists composition between class and students.
55.What are the advantages of Encapsulation in Java?
Ans:There are the following advantages of Encapsulation in Java?
- By providing only the setter or getter method, you can make the class read-only or write-only. In other words, you can skip the getter or setter methods.
- It provides you the control over the data. Suppose you want to set the value of id which should be greater than 100 only, you can write the logic inside the setter method. You can write the logic not to store the negative numbers in the setter methods.
- It is a way to achieve data hiding in Java because other class will not be able to access the data through the private data members.
- The encapsulate class is easy to test. So, it is better for unit testing.
- The standard IDE's are providing the facility to generate the getters and setters. So, it is easy and fast to create an encapsulated class in Java.
56.What is a lightweight component?
Ans:Lightweight components are the one which does not go with the native call to obtain the graphical units. They share their parent component graphical units to render them. For example, Swing components, and JavaFX Components.
57.What is object cloning?
Ans:The object cloning is a way to create an exact copy of an object. The clone() method of the Object class is used to clone an object. The java.lang.Cloneable interface must be implemented by the class whose object clone we want to create. If we don't implement Cloneable interface, clone() method generates CloneNotSupportedException. The clone() method is defined in the Object class. The syntax of the clone() method is as follows:
protected Object clone() throws CloneNotSupportedException
58.What are the advantages and disadvantages of object cloning?
Ans:Advantage of Object Cloning
- You don't need to write lengthy and repetitive codes. Just use an abstract class with a 4- or 5-line long clone() method.
- It is the easiest and most efficient way of copying objects, especially if we are applying it to an already developed or an old project. Just define a parent class, implement Cloneable in it, provide the definition of the clone() method and the task will be done.
- Clone() is the fastest way to copy the array.
Disadvantage of Object Cloning
- To use the Object.clone() method, we have to change many syntaxes to our code, like implementing a Cloneable interface, defining the clone() method and handling CloneNotSupportedException, and finally, calling Object.clone(), etc.
- We have to implement the Cloneable interface while it does not have any methods in it. We have to use it to tell the JVM that we can perform a clone() on our object.
- Object.clone() is protected, so we have to provide our own clone() and indirectly call Object.clone() from it.
- Object.clone() does not invoke any constructor, so we do not have any control over object construction.
- If you want to write a clone method in a child class, then all of its superclasses should define the clone() method in them or inherit it from another parent class. Otherwise, the super.clone() chain will fail.
- Object.clone() supports only shallow copying, but we will need to override it if we need deep cloning.
59.What is an applet?
Ans:An applet is a small java program that runs inside the browser and generates dynamic content. It is embedded in the webpage and runs on the client side. It is secured and takes less response time. It can be executed by browsers running under many platforms, including Linux, Windows, Mac Os, etc. However, the plugins are required at the client browser to execute the applet. The following image shows the architecture of Applet.
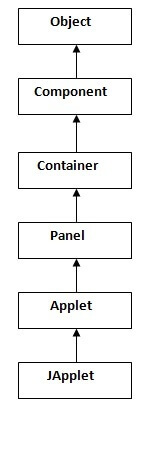
- init()
- start()
- paint()
- stop()
- destroy()
60.What is the difference between Synchronous programming and Asynchronous programming regarding a thread?
Ans:Synchronous programming: In Synchronous programming model, a thread is assigned to complete a task and hence thread started working on it, and it is only available for other tasks once it will end the assigned task.
Asynchronous Programming: In Asynchronous programming, one job can be completed by multiple threads and hence it provides maximum usability of the various threads.
61.How is the safety of a thread achieved?
Ans:If a method or class object can be used by multiple threads at a time without any race condition, then the class is thread-safe. Thread safety is used to make a program safe to use in multithreaded programming. It can be achieved by the following ways:
- Synchronization
- Using Volatile keyword
- Using a lock based mechanism
- Use of atomic wrapper classes
62.What are the main differences between array and collection?
Ans:Array and Collection are somewhat similar regarding storing the references of objects and manipulating the data, but they differ in many ways. The main differences between the array and Collection are defined below:
- Arrays are always of fixed size, i.e., a user can not increase or decrease the length of the array according to their requirement or at runtime, but In Collection, size can be changed dynamically as per need.
- Arrays can only store homogeneous or similar type objects, but in Collection, heterogeneous objects can be stored.
- Arrays cannot provide the ready-made methods for user requirements as sorting, searching, etc. but Collection includes ready-made methods to use.
63.How do you ensure that N thread can access N resources without deadlock?
Ans:The key point here is ordering, if you acquire resources in a particular order and release resources in the reverse order you can prevent deadlock.
64. What will be the Output of the below code:
public class A {
public static void main(String[] args)
{
if (true)
break;
}
}
Ans:Error
Reason: Break statement can only be used with loop or switch. So, using break with if statement causes “break outside switch or loop” error.
65.Can an application have multiple classes having main method?
Ans:Yes it is possible. While starting the application we mention the class name to be run. The JVM will look for the Main method only in the class whose name you have mentioned. Hence there is not conflict amongst the multiple classes having main method.
66.Can we import same package/class twice? Will the JVM load the package twice at runtime?
Ans:One can import the same package or same class multiple times. Neither compiler nor JVM complains about it. And the JVM will internally load the class only once no matter how many times you import the same class.
67.What is defined as an immutable object? How would you create an immutable object in Java?
Ans: Immutable objects are defined as those whose state cannot be changed once it has been made, any alteration will result in a new object, e.g. String, Integer, and other wrapper class.
68.What are the default values of Java primitives?
Ans:byte – 0, int – 0, boolean – false, short – 0, long – 0L, float – 0.0f, double – 0.0d, char – ‘\u0000’
69.What is a compile time constant in Java? What is the risk of using it?
Ans:Public static final variables are also known as the compile time constant, the public is optional there. They are substituted with actual values at compile time because compiler recognises their value up-front, and also recognise that it cannot be altered during runtime.
One of the issues is that if you choose to use a public static final variable from in-house or a third party library, and their value changed later, then your client will still be using the old value even after you deploy a new version of JARs.
This can be avoided by ensuring you compile your program when you upgrade dependency JAR files.
70.What is the difference between poll() and remove() method?
Ans:Both poll() and remove() take out the object from the Queue but if poll() fails, then it returns null. However, if remove() fails, it throws exception.
71.List listeners and their corresponding Methods.
Ans:ActionListerner – actionPerformed();
ItemListerner – itemStateChanged();TextListener – textValueChanged();
FocusListener – focusLost(); & FocusGained();
WindowListener – windowActified(); windowDEactified(); windowIconified(); windowDeiconified(); windowClosed(); windowClosing(); windowOpened();
MouseMotionListener – mouseDragged(); & mouseMoved();
MouseListener – mousePressed(); mouseReleased(); mouseEntered(); mouseExited(); mouseClicked();
72.What is Event handling in Java?
Ans:Is irrespective of any component, if any action performed/done on Frame, Panel or on window, handling those actions are called Event Handling.
73.What is JSP?
Ans:JSP is an abbreviation for Java Servlet Page. It is a Server-Side Programming Language used to create dynamic web-pages in the form of HTML. The JSP page is implicitly converted into a servlet and it enables some additional features such as Expression Language, Custom Tags, and many more.
74.What are the Literals used in JSP?
Ans:The Literals used in JSP are as follows:
- Null
- Boolean
- String
- Integer
- Float
75.Mention the Implicit Objects in a JSP.
Ans:The Web Container creates certain objects that include the information related to a particular Request, Application or a Page. These Objects are called as Implicit Objects. The Implicit Objects in the JSP are as follows:
- Request
- Response
- Application
- Exception
- Config
- Page
- Session
- PageContext
- Out
76. Can you stop Multiple Submits to a Web Page that are initiated by clicking to refresh button?
Ans:Yes, This issue can be solved by using a Post/Redirect/Get or a PRG pattern.
- A form filed by the user gets submitted to the server using POST/GET method.
- The state in the database and business model are updated.
- A redirect response is used to reply by the servlet for a view page.
- A view is loaded by the browser using the GET command and no user data is sent.
- This is safe from multiple submits as it is a separate JSP page.
77.How do you check if two given String are anagrams?
Ans: Anagrams are a mix-up of characters in String e.g. army and mary, stop and pots etc. To identify if Strings are anagram, you will need to get their character array and identify if they are equal or not.
You are able to use indexOf(), substring() and StringBuffer or StringBuilder class to solve this question.
78.String class is defined under which package in Java?
Ans:The answer to this Java question is java.lang package.
79.What is the difference between
79.What is the difference between String s = "Test"
and String s = new String("Test")
? Which is better and why?
Ans:In general,
String s = "Test"
is more efficient to use than String s = new String("Test")
.
In the case of
String s = "Test"
, a String with the value “Test” will be created in the String pool. If another String with the same value is then created (e.g., String s2 = "Test"
), it will reference this same object in the String pool.
However, if you use
String s = new String("Test")
, in addition to creating a String with the value “Test” in the String pool, that String object will then be passed to the constructor of the String Object (i.e., new String("Test")
) and will create another String object (not in the String pool) with that value. Each such call will therefore create an additional String object (e.g., String s2 = new String("Test")
would create an addition String object, rather than just reusing the same String object from the String pool).80.What Is the Difference Between Dynamic Binding and Static Binding?
Ans:Binding in Java is a process of associating a method call with the proper method body. We can distinguish two types of binding in Java: static and dynamic.
The main difference between static binding and dynamic binding is that static binding occurs at compile time and dynamic binding at runtime.
Static binding uses class information for binding. It's responsible for resolving class members that are private or static and final methods and variables. Also, static binding binds overloaded methods.
Dynamic binding, on the other hand, uses object information to resolve bindings. That's why it's responsible for resolving virtual and overridden methods.
81.Is there a destructor in Java?
Ans:In Java, the garbage collector automatically deletes the unused objects to free up the memory. Developers have no need to mark the objects for deletion, which is error-prone. So it's sensible Java has no destructors available.
In case the objects hold open sockets, open files, or database connections, the garbage collector is not able to reclaim those resources. We can release the resources in close method and use try-finally syntax to call the method afterward before Java 7, such as the I/O classes FileInputStreamand FileOutputStream. As of Java 7, we can implement interface AutoCloseable and use try-with-resources statement to write shorter and cleaner code. But it's possible the API users forget to call the close method, so the finalize method and Cleaner class come into existence to act as the safety net. But please be cautioned they are not equivalent to the destructor.
It's not assured both the finalize method and Cleaner class will run promptly. They even get no chance to run before the JVM exits. Although we could call System.runFinalization to suggest that JVM run the finalize methods of any objects pending for finalization, it's still non-deterministic.
Moreover, the finalize method can cause performance issues, deadlocks etc. We can find more information by looking at one of our articles: A Guide to the finalize Method in Java.
As of Java 9, Cleaner class is added to replace the finalize method because of the downsides it has. As a result, we have better control over the thread which does the cleaning actions.
But the java spec points out the behavior of cleaners during System.exit is implementation specific and Java provides no guarantees whether cleaning actions will be invoked or not.
82.Explain in brief the history of Java?
Ans:In the year 1991, a small group of engineers called ‘Green Team’ led by James Gosling, worked a lot and introduced a new programming language called “Java”. This language is created in such a way that it is going to revolutionize the world.
In today’s World, Java is not only invading the internet, but also it is an invisible force behind many of the operations, devices, and applications.
83.What does JVM do?
Ans:The JVM performs following operation:
- Loads code
- Verifies code
- Executes code
- Provides runtime environment
JVM provides definitions for the:
- Memory area
- Class file format
- Register set
- Garbage-collected heap
- Fatal error reporting etc.
84.Why Should We Use JDBC
Ans:Before JDBC, ODBC API was the database API to connect and execute the query with the database. But, ODBC API uses ODBC driver which is written in C language (i.e. platform dependent and unsecured). That is why Java has defined its own API (JDBC API) that uses JDBC drivers (written in Java language).
We can use JDBC API to handle database using Java program and can perform the following activities:
- Connect to the database
- Execute queries and update statements to the database
- Retrieve the result received from the database.
85.Java Networking Terminology
Ans:
- IP Address
- Protocol
- Port Number
- MAC Address
- Connection-oriented and connection-less protocol
- Socket
1) IP Address
IP address is a unique number assigned to a node of a network e.g. 192.168.0.1 . It is composed of octets that range from 0 to 255.
It is a logical address that can be changed.
2) Protocol
A protocol is a set of rules basically that is followed for communication. For example:
- TCP
- FTP
- Telnet
- SMTP
- POP etc.
3) Port Number
The port number is used to uniquely identify different applications. It acts as a communication endpoint between applications.
The port number is associated with the IP address for communication between two applications.
4) MAC Address
MAC (Media Access Control) Address is a unique identifier of NIC (Network Interface Controller). A network node can have multiple NIC but each with unique MAC.
5) Connection-oriented and connection-less protocol
In connection-oriented protocol, acknowledgement is sent by the receiver. So it is reliable but slow. The example of connection-oriented protocol is TCP.
But, in connection-less protocol, acknowledgement is not sent by the receiver. So it is not reliable but fast. The example of connection-less protocol is UDP.
6) Socket
A socket is an endpoint between two way communication.
Visit next page for java socket programming.
86.java.net package
Ans:The java.net package provides many classes to deal with networking applications in Java. A list of these classes is given below:
- Authenticator
- CacheRequest
- CacheResponse
- ContentHandler
- CookieHandler
- CookieManager
- DatagramPacket
- DatagramSocket
- DatagramSocketImpl
- InterfaceAddress
- JarURLConnection
- MulticastSocket
- InetSocketAddress
- InetAddress
- Inet4Address
- Inet6Address
- IDN
- HttpURLConnection
- HttpCookie
- NetPermission
- NetworkInterface
- PasswordAuthentication
- Proxy
- ProxySelector
- ResponseCache
- SecureCacheResponse
- ServerSocket
- Socket
- SocketAddress
- SocketImpl
- SocketPermission
- StandardSocketOptions
- URI
- URL
- URLClassLoader
- URLConnection
- URLDecoder
- URLEncoder
- URLStreamHandler
Comments
Post a Comment